First, you must download and install Windump.
http://www.winpcap.org/windump/default.htm
Create a new Windows forms project in Visual Studio 2008.
Add a textbox and a couple buttons to look like this:
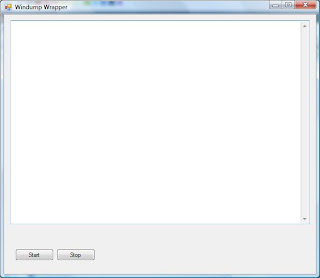
Copy code below into the Form1.cs file.
Change the "process.StartInfo.Filename" to wherever you saved windump. Also, don't forget to set the "process.StartInfo.Arguments" to your ethernet interface. On my PC it is interface number 2. For a complete list of possible arguments, see the Windump Docs.
http://www.winpcap.org/windump/docs/manual.htm
Download the source WindumpWrapper.zip
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.Reflection;
using System.IO;
using System.Diagnostics;
using System.Threading;
namespace WindumpWrapper
{
public partial class Form1 : Form
{
private delegate void WindumpEventHandler(string data);
private System.Diagnostics.Process process = null;
public Form1()
{
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e)
{
}
public void StartWinDump()
{
try
{
//Get a list of all the processes
Process[] processlist = Process.GetProcesses();
//Kill any existing windump processes
foreach (Process theprocess in processlist)
{
if (theprocess.ProcessName == "WinDump")
{
theprocess.Kill();
}
}
//process doesn't exist so create it
process = new System.Diagnostics.Process();
process.StartInfo.FileName = "C:\\Program Files (x86)\\WinPcap\\WinDump.exe";
//Set the arguments here, such as interface number
process.StartInfo.Arguments = "-i2";
process.StartInfo.CreateNoWindow = true;
process.StartInfo.UseShellExecute = false;
process.StartInfo.RedirectStandardOutput = true;
process.Start();
process.PriorityClass = System.Diagnostics.ProcessPriorityClass.Normal;
process.OutputDataReceived += new System.Diagnostics.DataReceivedEventHandler(process_OutputDataReceived);
process.BeginOutputReadLine();
}
catch (Exception ex)
{
LogError(ex);
}
}
void process_OutputDataReceived(object sender, System.Diagnostics.DataReceivedEventArgs e)
{
try
{
this.Invoke(new WindumpEventHandler(AddData),e.Data.ToString());
}
catch (Exception ex)
{
LogError(ex);
}
}
private void StopWinDump()
{
try
{
if (process != null)
{
if (process.ProcessName == "WinDump")
{
process.Kill();
}
}
}
catch (Exception ex)
{
LogError(ex);
}
}
private void LogError(Exception exp)
{
string appFullPath = Assembly.GetCallingAssembly().Location;
string logPath = appFullPath.Substring(0, appFullPath.LastIndexOf("\\")) + ".log";
StreamWriter writer = new StreamWriter(logPath, true);
try
{
writer.WriteLine(logPath,
String.Format("Error in Windump Wrapper: {0} \r\n StackTrace: {1}", exp.Message, exp.StackTrace));
}
catch { }
finally
{
writer.Close();
}
}
private void Form1_FormClosing(object sender, FormClosingEventArgs e)
{
StopWinDump();
textBox1.AppendText("Stopped...\n");
}
private void btnStart_Click(object sender, EventArgs e)
{
StartWinDump();
textBox1.AppendText("Starting...\n");
}
private void btnStop_Click(object sender, EventArgs e)
{
StopWinDump();
}
private void AddData(string data)
{
textBox1.AppendText(data);
}
}
}
No comments:
Post a Comment